Bài học này chúng ta tiếp tục làm việc với BarGraph, Tui hướng dẫn các bạn cách bổ sung thiết kế giao diện trong Qt Designer để cung cấp các chức năng tương tác trong quá trình trực quan hóa dữ liệu với BarGraph:
- Chức năng ẩn và hiện Background Grid
- Chức năng ẩn và hiện Legend
- Chức năng đổi màu nền của Chart dùng QColorDialog
- Chức năng đổi màu nền của Bar dùng QColorDialog
Các chức năng này đều được thiết kế giao diện và cung cấp sự kiện tương tác người dùng. Dựa vào bài này mà các bạn có thể tùy chỉnh tương tác biểu đồ trong các trường hợp thực tế khác nhau.
Ngoài ra các bạn cũng được ôn tập lại các kiến thức liên quan tới QCheckBox, xử lý các sự kiện trên QCheckBox. Cũng như xử lý sự kiện trên QPushButton.
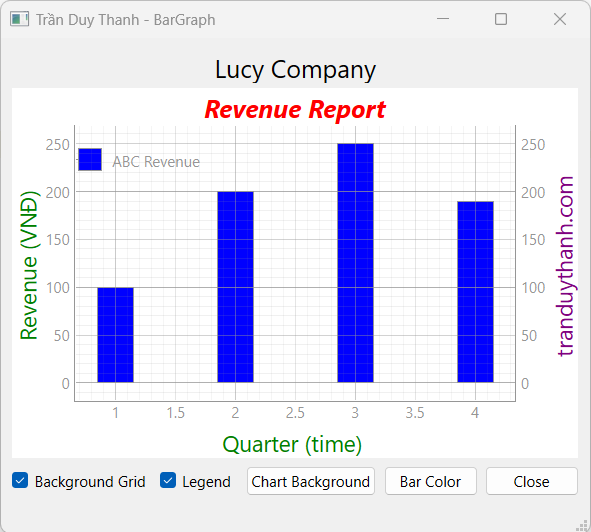
Bạn quan sát giao diện ở trên có 2 QCheckBox, 3 QPushButton được bổ sung vào giao diện để cho người dùng tương tác. Bài này mở rộng từ bài trước
Dưới đây là chi tiết từng bước thực hiện dự án:
Bước 1: Tạo dự án “LearnPyQtBarGraphPart4” trong Pycharm có cấu trúc như dưới đây:
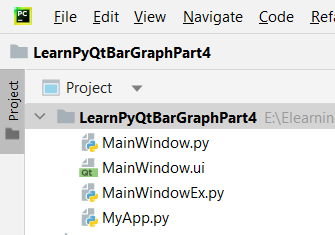
- “MainWindow.ui” là giao diện thiết kế tương tác người dùng bằng Qt Designer
- “MainWindow.py” là Generate Python code cho giao diện “MainWindow.ui”
- “MainWindowEx.py” là file mã lệnh kế thừa từ Generate Python Code để xử lý: Nạp giao diện, hiển thị chart, gán sự kiện và không bị lệ thuộc vào giao diện bị thay đổi sau này khi Generate lại code
- “MyApp.py” là file mã lệnh thực thi chương trình.
Bước 2: Thiết kế giao diện “MainWindow.ui” và đặt tên cho Widget/layout như hình dưới đây:
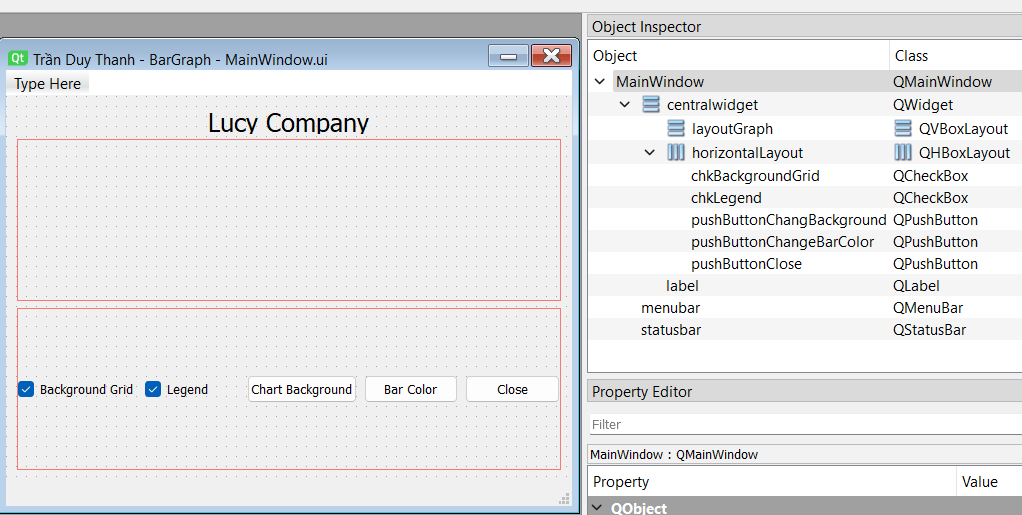
Bạn lần lượt kéo thả các Widget vào giao diện như trên, lưu ý việc lựa chọn các Layout cho phù hợp.
Bước 3: Generate Python Code cho “MainWindow.ui”, lúc này mã lệnh “MainWindow.py” tự động được tạo ra:
# Form implementation generated from reading ui file 'MainWindow.ui'
#
# Created by: PyQt6 UI code generator 6.5.2
#
# WARNING: Any manual changes made to this file will be lost when pyuic6 is
# run again. Do not edit this file unless you know what you are doing.
from PyQt6 import QtCore, QtGui, QtWidgets
class Ui_MainWindow(object):
def setupUi(self, MainWindow):
MainWindow.setObjectName("MainWindow")
MainWindow.resize(566, 436)
self.centralwidget = QtWidgets.QWidget(parent=MainWindow)
self.centralwidget.setObjectName("centralwidget")
self.verticalLayout_2 = QtWidgets.QVBoxLayout(self.centralwidget)
self.verticalLayout_2.setObjectName("verticalLayout_2")
self.label = QtWidgets.QLabel(parent=self.centralwidget)
sizePolicy = QtWidgets.QSizePolicy(QtWidgets.QSizePolicy.Policy.Preferred, QtWidgets.QSizePolicy.Policy.Fixed)
sizePolicy.setHorizontalStretch(0)
sizePolicy.setVerticalStretch(0)
sizePolicy.setHeightForWidth(self.label.sizePolicy().hasHeightForWidth())
self.label.setSizePolicy(sizePolicy)
self.label.setMinimumSize(QtCore.QSize(0, 25))
self.label.setMaximumSize(QtCore.QSize(16777215, 20))
font = QtGui.QFont()
font.setPointSize(15)
self.label.setFont(font)
self.label.setAlignment(QtCore.Qt.AlignmentFlag.AlignCenter)
self.label.setObjectName("label")
self.verticalLayout_2.addWidget(self.label)
self.layoutGraph = QtWidgets.QVBoxLayout()
self.layoutGraph.setSizeConstraint(QtWidgets.QLayout.SizeConstraint.SetDefaultConstraint)
self.layoutGraph.setObjectName("layoutGraph")
self.verticalLayout_2.addLayout(self.layoutGraph)
self.horizontalLayout = QtWidgets.QHBoxLayout()
self.horizontalLayout.setObjectName("horizontalLayout")
self.chkBackgroundGrid = QtWidgets.QCheckBox(parent=self.centralwidget)
self.chkBackgroundGrid.setChecked(True)
self.chkBackgroundGrid.setObjectName("chkBackgroundGrid")
self.horizontalLayout.addWidget(self.chkBackgroundGrid)
self.chkLegend = QtWidgets.QCheckBox(parent=self.centralwidget)
self.chkLegend.setChecked(True)
self.chkLegend.setObjectName("chkLegend")
self.horizontalLayout.addWidget(self.chkLegend)
self.pushButtonChangBackground = QtWidgets.QPushButton(parent=self.centralwidget)
self.pushButtonChangBackground.setObjectName("pushButtonChangBackground")
self.horizontalLayout.addWidget(self.pushButtonChangBackground)
self.pushButtonChangeBarColor = QtWidgets.QPushButton(parent=self.centralwidget)
self.pushButtonChangeBarColor.setObjectName("pushButtonChangeBarColor")
self.horizontalLayout.addWidget(self.pushButtonChangeBarColor)
self.pushButtonClose = QtWidgets.QPushButton(parent=self.centralwidget)
self.pushButtonClose.setObjectName("pushButtonClose")
self.horizontalLayout.addWidget(self.pushButtonClose)
self.verticalLayout_2.addLayout(self.horizontalLayout)
MainWindow.setCentralWidget(self.centralwidget)
self.menubar = QtWidgets.QMenuBar(parent=MainWindow)
self.menubar.setGeometry(QtCore.QRect(0, 0, 566, 22))
self.menubar.setObjectName("menubar")
MainWindow.setMenuBar(self.menubar)
self.statusbar = QtWidgets.QStatusBar(parent=MainWindow)
self.statusbar.setObjectName("statusbar")
MainWindow.setStatusBar(self.statusbar)
self.retranslateUi(MainWindow)
self.pushButtonClose.clicked.connect(MainWindow.close) # type: ignore
QtCore.QMetaObject.connectSlotsByName(MainWindow)
def retranslateUi(self, MainWindow):
_translate = QtCore.QCoreApplication.translate
MainWindow.setWindowTitle(_translate("MainWindow", "Trần Duy Thanh - BarGraph"))
self.label.setText(_translate("MainWindow", "Lucy Company"))
self.chkBackgroundGrid.setText(_translate("MainWindow", "Background Grid"))
self.chkLegend.setText(_translate("MainWindow", "Legend"))
self.pushButtonChangBackground.setText(_translate("MainWindow", "Chart Background"))
self.pushButtonChangeBarColor.setText(_translate("MainWindow", "Bar Color"))
self.pushButtonClose.setText(_translate("MainWindow", "Close"))
Bước 4: Viết mã lệnh cho “MainWindowEx.py”
Lớp MainWindowEx kế thừa lớp Ui_MainWindow (lớp được Generate Python code ở bước trước)
Tui định nghĩa 1 constructor __init__() gọi lại constructor ở lớp cha, tạm thời trong bài tập này chưa xử lý gì khác.
from PyQt6.QtCore import Qt
from PyQt6.QtWidgets import QColorDialog
from MainWindow import Ui_MainWindow
import numpy as np
import pyqtgraph as pg
class MainWindowEx(Ui_MainWindow):
def __init__(self):
super().__init__()
def setupUi(self, MainWindow):
super().setupUi(MainWindow)
self.MainWindow=MainWindow
self.setupBarGraph()
Hàm setupUi() được override để nạp giao diện, lưu lại biến MainWindow để sử dụng cho quá trình xử lý trong tương lai. Đồng thời nó cũng gọi hàm setupBarGraph() để hiển thị Chart, Hàm này ta viết như sau:
def setupBarGraph(self):
self.graphWidget = pg.PlotWidget()
self.graphWidget.setTitle("Revenue Report", color="r", size="15pt", bold=True, italic=True)
self.graphWidget.setBackground('w')
labelStyle = {"color": "green", "font-size": "18px"}
labelBrandStyle = {"color": "purple", "font-size": "18px"}
self.graphWidget.setLabel("left","Revenue (VNĐ)",**labelStyle)
self.graphWidget.setLabel("bottom","Quarter (time)",**labelStyle)
self.graphWidget.setLabel("right", "tranduythanh.com", **labelBrandStyle)
self.graphWidget.showGrid(x=True, y=True)
width = 0.3
quarter = np.array([1, 2, 3, 4])
revenue = [100, 200, 250, 190]
self.bargraphItem = pg.BarGraphItem(x=quarter, height=revenue, width=width, brush='b', name="ABC Revenue")
self.legend=self.graphWidget.addLegend()
self.graphWidget.addItem(self.bargraphItem)
self.layoutGraph.addWidget( self.graphWidget)
self.chkBackgroundGrid.stateChanged.connect(self.processChangeGrid)
self.chkLegend.stateChanged.connect(self.processChangeLegend)
self.pushButtonChangBackground.clicked.connect(self.processChangeChartBackground)
self.pushButtonChangeBarColor.clicked.connect(self.processChangeBarColor)
- Tất cả các kỹ thuật liên quan tới PlotWidget và cách xử lý BarGraphItem Tui đã trình bày chi tiết và cụ thể ở các bài trước rồi, nên bài này Tui không nhắc lại. Mà chúng ta chỉ quan tâm xử lý Signal liên quan tới các QCheckBox và QPushButton để cung cấp chức năng tương tác Chart cho người dùng.
- Ta xem Signal statechanged của QCheckBox background grid:
self.chkBackgroundGrid.stateChanged.connect(self.processChangeGrid)
Slot(hàm) chi tiết xử lý khi người dùng Checked vào QCheckBox background grid được khai báo trong hàm processChangeGrid():
def processChangeGrid(self,value):
state=Qt.CheckState(value)
if state==Qt.CheckState.Checked:
self.graphWidget.showGrid(x=True, y=True)
else:
self.graphWidget.showGrid(x=False, y=False)
Khi chạy chức năng này thì ta thấy như sau: Nếu người sử dụng Unchecked QCheckBox background Grid này thì các lưới sẽ bị biến mất, còn Checked thì các lưới sẽ được hiển thị trở lại:
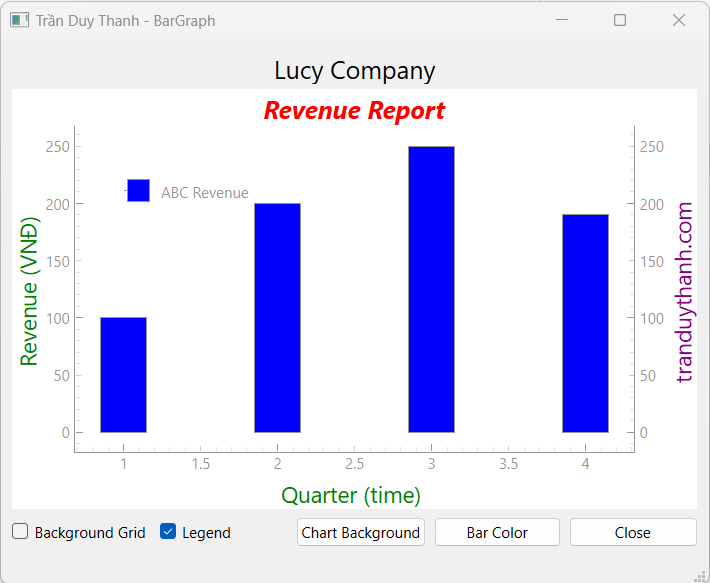
- Tiếp theo Ta xem Signal statechanged của QCheckBox Legend:
self.chkLegend.stateChanged.connect(self.processChangeLegend)
Slot(hàm) chi tiết xử lý khi người dùng Checked vào QCheckBox legend được khai báo trong hàm processChangeLegend():
def processChangeLegend(self,value):
state=Qt.CheckState(value)
if state==Qt.CheckState.Checked:
self.legend.show()
else:
self.legend.hide()
Khi chạy chức năng này thì ta thấy như sau: Nếu người sử dụng Unchecked QCheckBox Legend này thì Legend bị biến mất, còn Checked thì Legend sẽ được hiển thị trở lại:
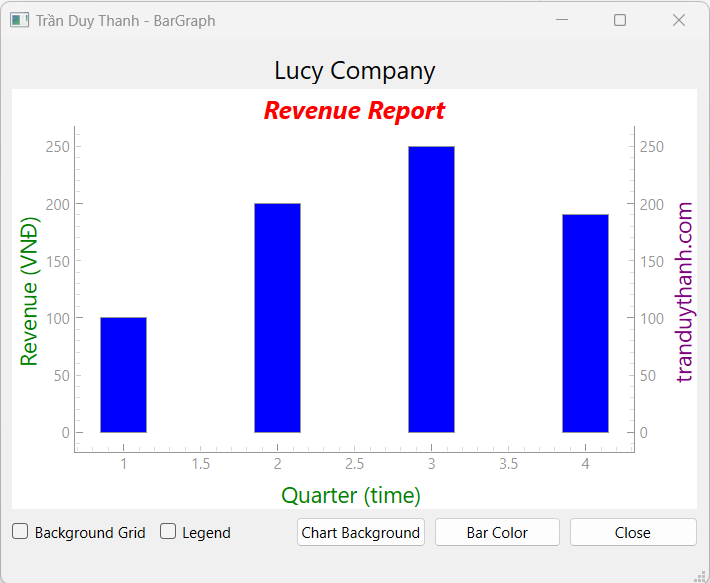
- Tiếp theo ta qua Signal clicked cho QPushButton thay đổi màu nền của Chart:
self.pushButtonChangBackground.clicked.connect(self.processChangeChartBackground)
Slot(hàm) chi tiết xử lý khi người dùng nhấn vào QPushButton Chart Background được khai báo trong hàm processChangeChartBackground():
def processChangeChartBackground(self):
dialog = QColorDialog()
if dialog.exec():
color = dialog.currentColor()
self.graphWidget.setBackground(color.name())
del dialog
Ta thấy, hàm trên Tui dùng QColorDialog() để hiển thị màn hình chọn màu, khi người dùng lựa chọn màu và nhần OK thì màu sẽ được lấy từ hàm currentColor(), sau đó ta truy suất tên của màu này để đổi màu cho Chart thông qua hàm: setBackground(color.name()).
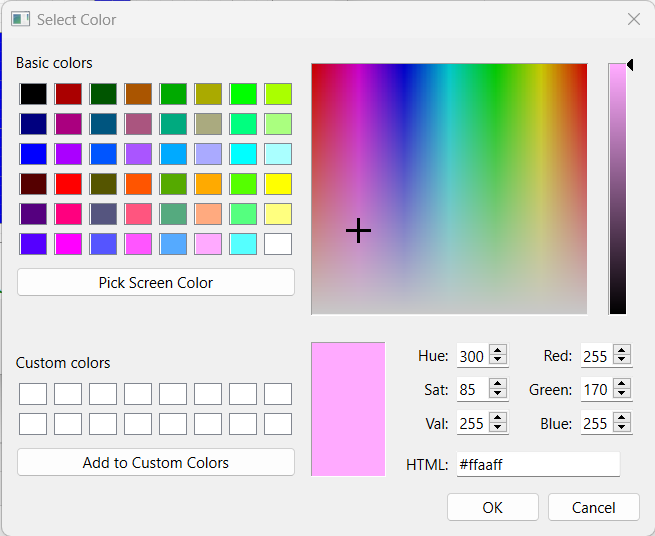
Ví dụ người dùng chọn màu hồng rồi nhấn OK. Lúc này nền màu của Chart sẽ đổi thành màu hồng này:
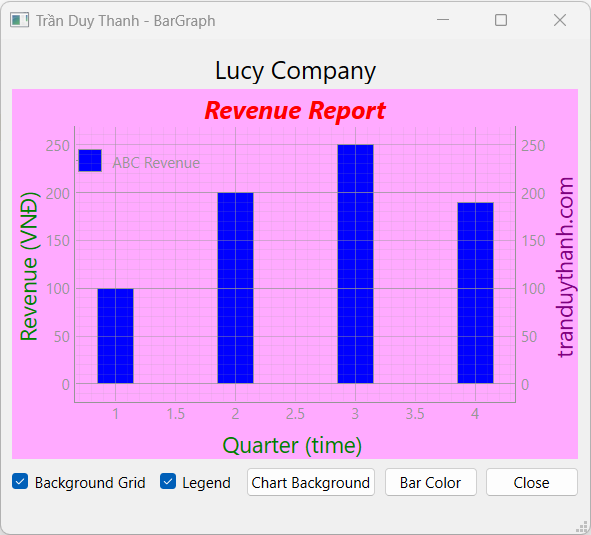
- Tiếp theo ta qua Signal clicked cho QPushButton thay đổi màu của Bar:
self.pushButtonChangeBarColor.clicked.connect(self.processChangeBarColor)
Slot(hàm) chi tiết xử lý khi người dùng nhấn vào QPushButton Chart Background được khai báo trong hàm processChangeBarColor():
def processChangeBarColor(self):
dialog = QColorDialog()
if dialog.exec():
color = dialog.currentColor()
self.bargraphItem.opts["brush"]=color.name()
self.bargraphItem._updateColors(self.bargraphItem.opts)
del dialog
Mã lệnh ở trên Tui cũng sử dụng QColorDialog để cho người dùng lựa chọn màu, sau khi lựa chọn màu thì tên màu sẽ được lưu với mảng opts[“brush”] của BarGraphItem.
sau đó nó sẽ được cập nhật màu thông qua hàm:
_updateColors(self.bargraphItem.opts) của BarGraphItem
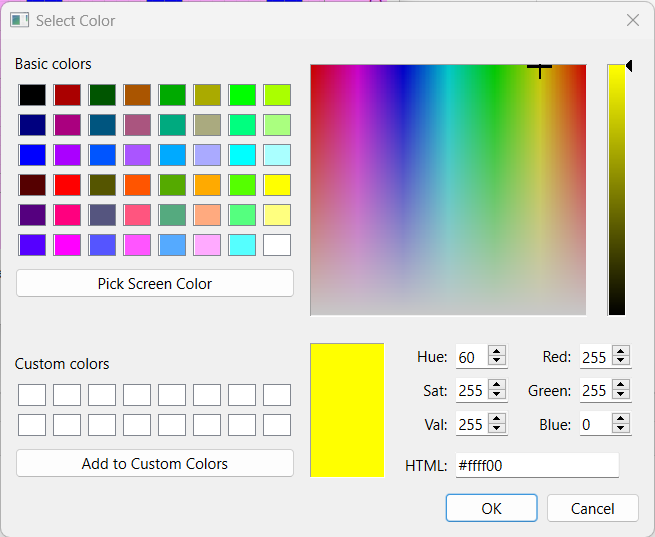
Ví dụ người dùng chọn màu vàng rồi nhấn OK:
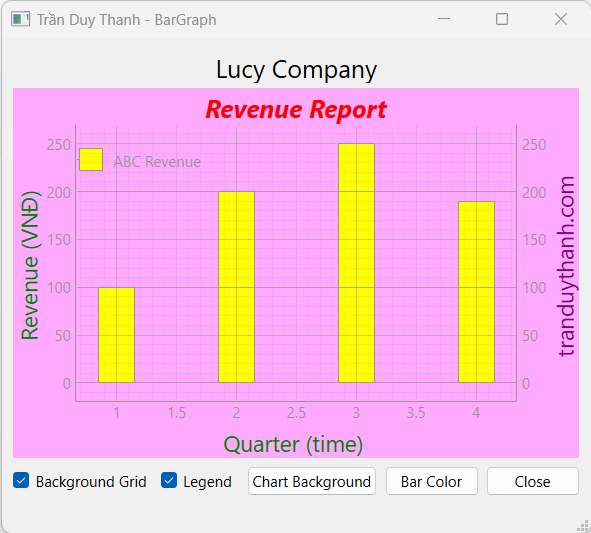
Dưới đây là mã lệnh đầy đủ của “MainWindowEx.py“:
from PyQt6.QtCore import Qt
from PyQt6.QtWidgets import QColorDialog
from MainWindow import Ui_MainWindow
import numpy as np
import pyqtgraph as pg
class MainWindowEx(Ui_MainWindow):
def __init__(self):
super().__init__()
def setupUi(self, MainWindow):
super().setupUi(MainWindow)
self.MainWindow=MainWindow
self.setupBarGraph()
def setupBarGraph(self):
self.graphWidget = pg.PlotWidget()
self.graphWidget.setTitle("Revenue Report", color="r", size="15pt", bold=True, italic=True)
self.graphWidget.setBackground('w')
labelStyle = {"color": "green", "font-size": "18px"}
labelBrandStyle = {"color": "purple", "font-size": "18px"}
self.graphWidget.setLabel("left","Revenue (VNĐ)",**labelStyle)
self.graphWidget.setLabel("bottom","Quarter (time)",**labelStyle)
self.graphWidget.setLabel("right", "tranduythanh.com", **labelBrandStyle)
self.graphWidget.showGrid(x=True, y=True)
width = 0.3
quarter = np.array([1, 2, 3, 4])
revenue = [100, 200, 250, 190]
self.bargraphItem = pg.BarGraphItem(x=quarter, height=revenue, width=width, brush='b', name="ABC Revenue")
self.legend=self.graphWidget.addLegend()
self.graphWidget.addItem(self.bargraphItem)
self.layoutGraph.addWidget( self.graphWidget)
self.chkBackgroundGrid.stateChanged.connect(self.processChangeGrid)
self.chkLegend.stateChanged.connect(self.processChangeLegend)
self.pushButtonChangBackground.clicked.connect(self.processChangeChartBackground)
self.pushButtonChangeBarColor.clicked.connect(self.processChangeBarColor)
def processChangeGrid(self,value):
state=Qt.CheckState(value)
if state==Qt.CheckState.Checked:
self.graphWidget.showGrid(x=True, y=True)
else:
self.graphWidget.showGrid(x=False, y=False)
def processChangeLegend(self,value):
state=Qt.CheckState(value)
if state==Qt.CheckState.Checked:
self.legend.show()
else:
self.legend.hide()
def processChangeChartBackground(self):
dialog = QColorDialog()
if dialog.exec():
color = dialog.currentColor()
self.graphWidget.setBackground(color.name())
del dialog
def processChangeBarColor(self):
dialog = QColorDialog()
if dialog.exec():
color = dialog.currentColor()
self.bargraphItem.opts["brush"]=color.name()
self.bargraphItem._updateColors(self.bargraphItem.opts)
del dialog
def show(self):
self.MainWindow.show()
Bước 5: Viết mã lệnh “MyApp.py” để thực thi chương trình:
from PyQt6.QtWidgets import QApplication, QMainWindow
from MainWindowEx import MainWindowEx
app=QApplication([])
myWindow=MainWindowEx()
myWindow.setupUi(QMainWindow())
myWindow.show()
app.exec()
Chạy “MyApp.py” ta có kết quả như mong muốn, các biểu đồ cột, các nhãn của các cột, tiêu đề chart, các QCheckBox và QPushButton được hiển thị để người dùng tương tác:
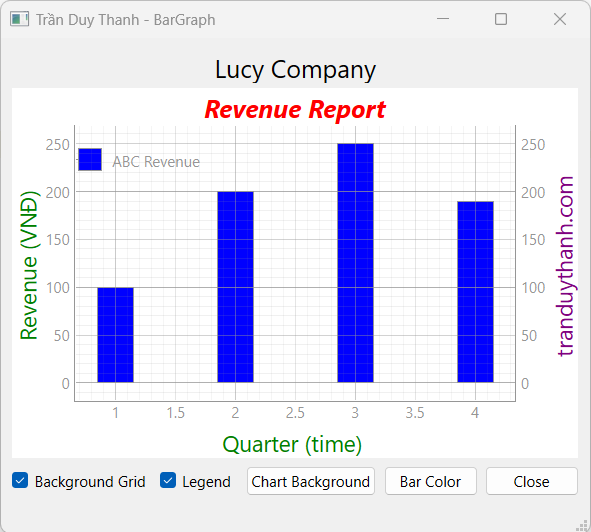
Như vậy tới đây Tui đã trình bày xong cách lập trình xử lý trực quan hóa dữ liệu với BarGraph. Ôn tập lại cách khai báo các mảng dữ liệu tương ứng với các trục, Ôn tập cách gọi các hàm liên quan tới BarGraphItem để hiển thị Chart. Cũng như ôn tập lại được toàn bộ kiến thức liên quan tới PlotWidget. Đặc biệt các bạn biết cách tương tác Chart thông qua các QCheckBox và QPushButton để tương tác lưới của chart, tương tác legend, đổi màu chart và màu bar.
Soure code của bài này các bạn tải ở đây:
https://www.mediafire.com/file/mrb3yyoajnirgfr/LearnPyQtBarGraphPart4.rar/file
Bài học sau Tui sẽ hướng dẫn các bạn cách lập trình để hiển thị ticks và cách tự động gán notation cho từng Bar, các bạn chú ý theo dõi
Chúc các bạn thành công
One thought on “Bài 34: Trực quan hóa dữ liệu – PyQtGraph-BarGraph-PyQt6 – Part 4”