Ở bài 5, Tui đã hướng dẫn dẫn các bạn cách tạo API lấy danh sách employees rồi, trong quá trình truy vấn dữ liệu, đôi khi ta cần sắp xếp dữ liệu. Ở bài này Tui sẽ trình bày cách sắp xếp dữ liệu (tăng dần hoặc giảm dần tùy thuộc vào người dùng). Lưu ý dữ liệu ở đây là Fake, Tui tạo một số dữ liệu để Test, ở các bài sau chúng ta sẽ dùng dữ liệu trực tiếp trên MongoDB. Các bài này ở mức cơ bản, mục đích là giúp các bạn biết được kỹ thuật lấy danh sách cũng như lấy parameter từ chỗ gọi API như thế nào, khi qua MongoDB thì kỹ thuật y chang, chỉ khác chỗ thay vì lấy dữ liệu giả thì lấy dữ liệu thật từ MongoDB thôi.
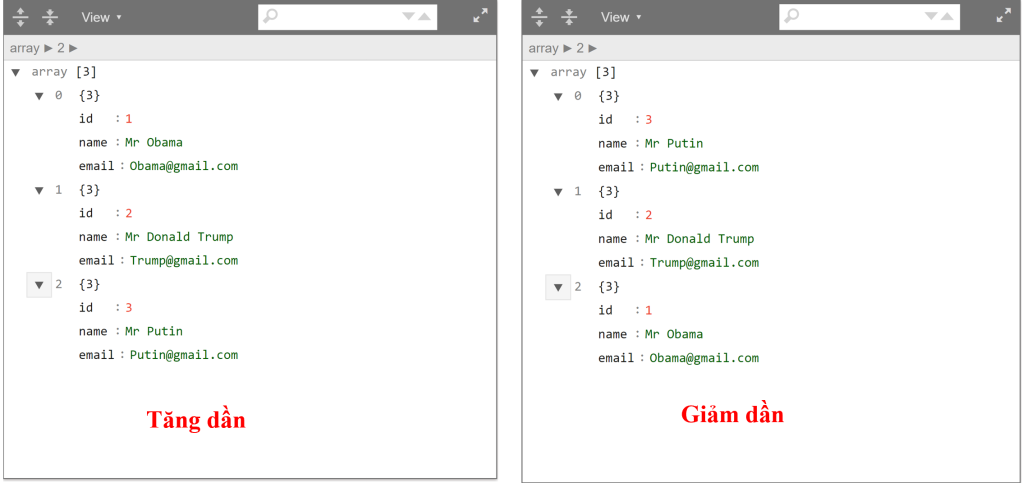
Ta tiếp tục mở lại dự án “LearnRESTApi“. Trong EmployeeVerticle bổ sung thêm hàm getSortEmployees có nội dung như dưới đây:
[code language=”java”]
/**
* Hàm này dùng để lấy danh sách dữ liệu Employee mà có sắp xếp
* http://localhost:8080/api/employeessort?sort=desc ->giảm dần
* http://localhost:8080/api/employeessort?sort=asc ->tăng dần
* @param routingContext
*/
private void getSortEmployees(RoutingContext routingContext) {
HttpServerResponse response=routingContext.response();
response.putHeader(“content-type”,”application/json;charset=UTF-8″);
//parameter lấy từ người dùng
String sort = routingContext.request().getParam(“sort”);
if (sort == null)
{
//nếu không có thì cho lỗi luôn API
routingContext.response().setStatusCode(400).end();
}
else
{
//dùng ArrayList để lưu trữ các Key của dữ liệu
ArrayList sortedKeys =
new ArrayList(employees.keySet());
//mặc định sắp xếp tăng dần các Key
Collections.sort(sortedKeys);
//nếu sort là desc (giảm dần)
if(sort.equalsIgnoreCase(“desc”))
{
//thì đảo ngược lại danh sách đang tăng dần -> nó tự thành giảm dần
Collections.reverse(sortedKeys);
}
//khai báo danh sách Employee là ArrayList
ArrayListsortEmployees=new ArrayList();
//vòng lặp theo Key
for (int key : sortedKeys)
{
//mỗi lần lấy employees.get(key) là đã lấy tăng dần hoặc giảm dần (vì key đã sắp xếp)
sortEmployees.add(employees.get(key));
}
//trả về danh sách đã sắp xêp
response.end(Json.encodePrettily(sortEmployees));
}
}
[/code]
Trong hàm start bổ sung thêm lệnh:
router.get(“/api/employeessort”).handler(this::getSortEmployees);
mục đích lệnh trên là tạo ra API tên là employeessort
Code chi tiết hàmg start sau khi sửa:
[code language=”java”]
@Override
public void start(Promise startPromise) throws Exception {
createExampleData();
Router router=Router.router(vertx);
router.get(“/api/employees”).handler(this::getAllEmployees);
router.get(“/api/employeessort”).handler(this::getSortEmployees);
vertx
.createHttpServer()
.requestHandler(router::accept)
.listen(config().getInteger(“http.port”, 8080),
result -> {
if (result.succeeded()) {
startPromise.complete();
} else {
startPromise.fail(result.cause());
}
}
);
}
[/code]
Dưới đây là coding đầy đủ của EmployeeVerticle:
[code language=”java”]
package tranduythanh.com.verticle;
import io.vertx.core.AbstractVerticle;
import io.vertx.core.Promise;
import io.vertx.core.http.HttpServerResponse;
import io.vertx.core.json.Json;
import io.vertx.ext.web.Router;
import io.vertx.ext.web.RoutingContext;
import tranduythanh.com.model.Employee;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
public class EmployeeVerticle extends AbstractVerticle
{
private HashMap employees=new HashMap();
public void createExampleData()
{
employees.put(1,new Employee(1,”Mr Obama”,”Obama@gmail.com”));
employees.put(2,new Employee(2,”Mr Donald Trump”,”Trump@gmail.com”));
employees.put(3,new Employee(3,”Mr Putin”,”Putin@gmail.com”));
}
private void getAllEmployees(RoutingContext routingContext) {
HttpServerResponse response=routingContext.response();
response.putHeader(“content-type”,”application/json;charset=UTF-8″);
response.end(Json.encodePrettily(employees.values()));
}
@Override
public void start(Promise startPromise) throws Exception {
createExampleData();
Router router=Router.router(vertx);
router.get(“/api/employees”).handler(this::getAllEmployees);
router.get(“/api/employeessort”).handler(this::getSortEmployees);
vertx
.createHttpServer()
.requestHandler(router::accept)
.listen(config().getInteger(“http.port”, 8080),
result -> {
if (result.succeeded()) {
startPromise.complete();
} else {
startPromise.fail(result.cause());
}
}
);
}
/**
* Hàm này dùng để lấy danh sách dữ liệu Employee mà có sắp xếp
* http://localhost:8080/api/employeessort?sort=desc ->giảm dần
* http://localhost:8080/api/employeessort?sort=asc ->tăng dần
* @param routingContext
*/
private void getSortEmployees(RoutingContext routingContext) {
HttpServerResponse response=routingContext.response();
response.putHeader(“content-type”,”application/json;charset=UTF-8″);
//parameter lấy từ người dùng
String sort = routingContext.request().getParam(“sort”);
if (sort == null)
{
//nếu không có thì cho lỗi luôn API
routingContext.response().setStatusCode(400).end();
}
else
{
//dùng ArrayList để lưu trữ các Key của dữ liệu
ArrayList sortedKeys =
new ArrayList(employees.keySet());
//mặc định sắp xếp tăng dần các Key
Collections.sort(sortedKeys);
//nếu sort là desc (giảm dần)
if(sort.equalsIgnoreCase(“desc”))
{
//thì đảo ngược lại danh sách đang tăng dần -> nó tự thành giảm dần
Collections.reverse(sortedKeys);
}
//khai báo danh sách Employee là ArrayList
ArrayListsortEmployees=new ArrayList();
//vòng lặp theo Key
for (int key : sortedKeys)
{
//mỗi lần lấy employees.get(key) là đã lấy tăng dần hoặc giảm dần (vì key đã sắp xếp)
sortEmployees.add(employees.get(key));
}
//trả về danh sách đã sắp xêp
response.end(Json.encodePrettily(sortEmployees));
}
}
}
[/code]
Lưu ý, khi chỉnh sửa code thì cần biên dịch lại các Verticle, nếu không có thể nó vẫn chạy code cũ:
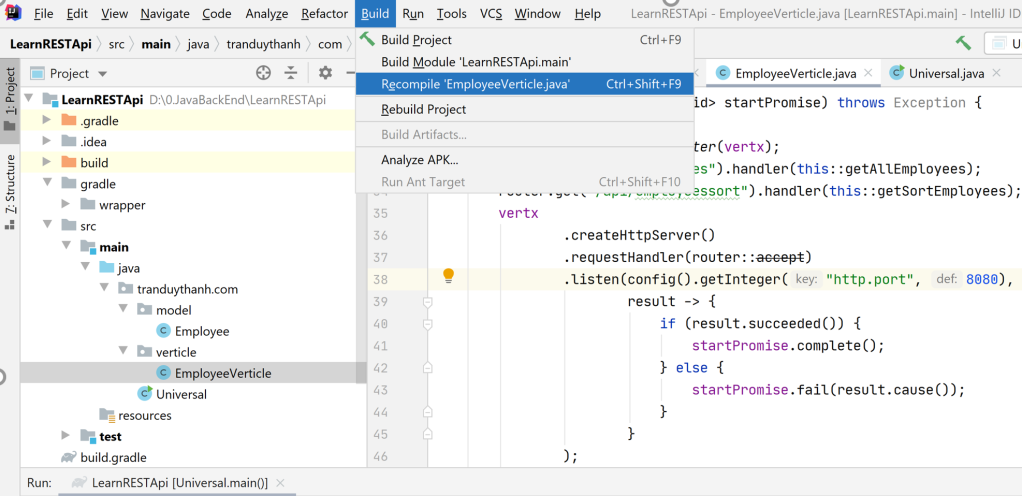
Ta mở class EmployeeVerticle–> vào menu Build –>chọn Recompile ‘EmployeeVerticle.java‘ hoặc nhấn tổ hợp phím tắt “Ctrl+Shift+F9“
Sau đó mới chạy Universal:
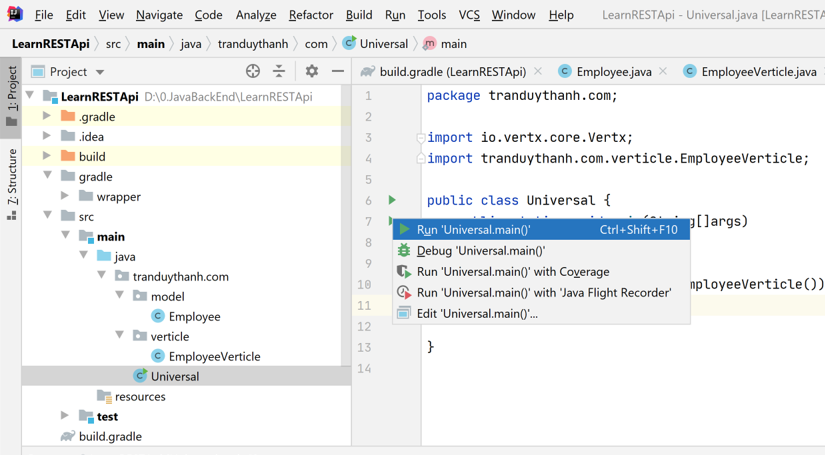
Sau khi chạy xong thì ta có thể test các API:
- lấy danh sách Employee giảm dần: http://localhost:8080/api/employeessort?sort=desc
- lấy danh sách Employee tăng dần: http://localhost:8080/api/employeessort?sort=asc
Minh họa gọi API xem danh sách Employee giảm dần theo id:
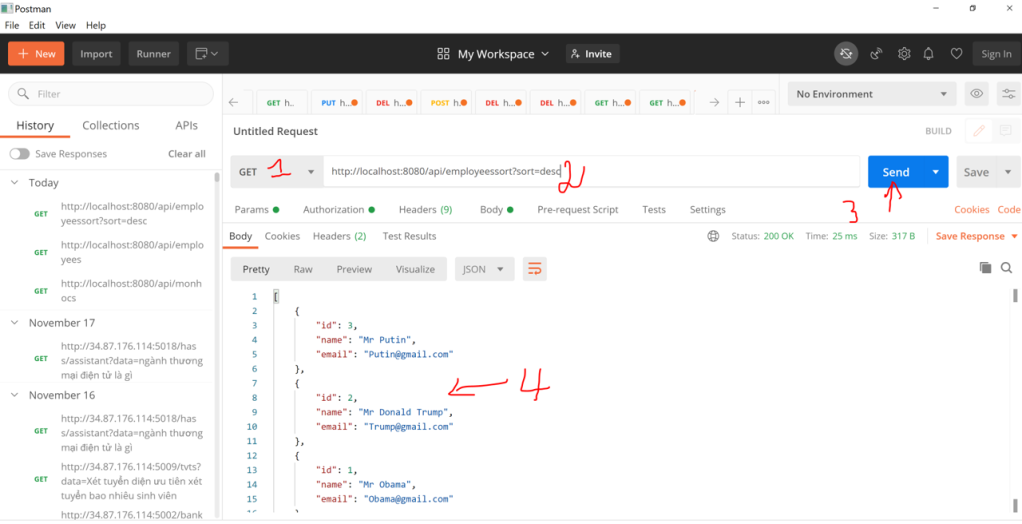
Minh họa gọi API xem danh sách Employee tăng dần theo id:
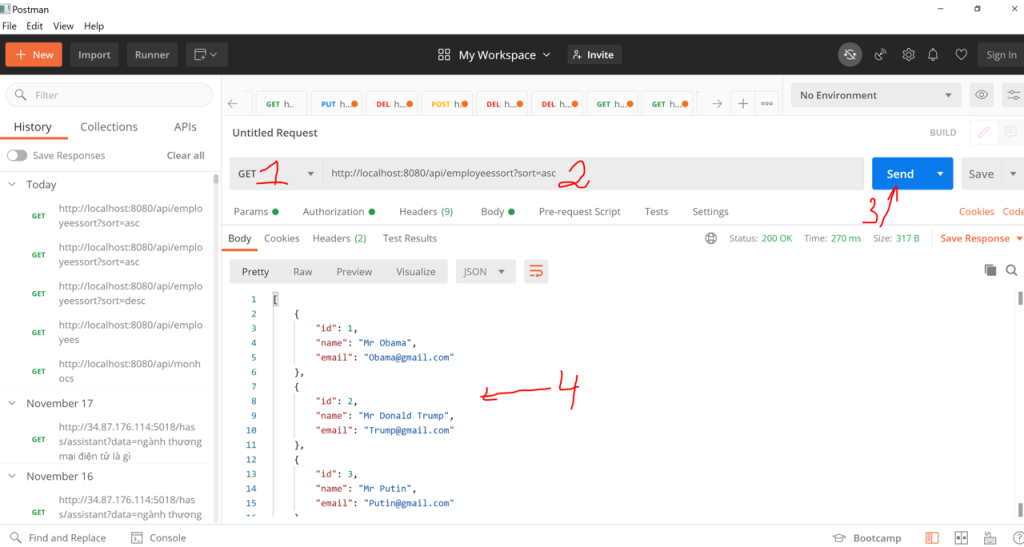
Ngoài ra với các API mà có các Parameter truyền vào, ta thường xuyên phải dùng chức năng debug để xem giá trị họ truyền vào có đúng hay chưa?
ta tạo BreakPoint cho nó trước (breakpoint là điểm mà phần mềm sẽ dừng lại để cho ta review tiến trình chạy). Ví dụ ta muốn xem hàm sắp xếp nó lấy parameter và truy vấ dữ liệu thì ta cần tạo breakpoint –>bằng cách click chuột vào vị trí mũi tên màu đỏ ở dòng mà ta muốn debug–>nó ra điểm dừng hình tròn màu đỏ.
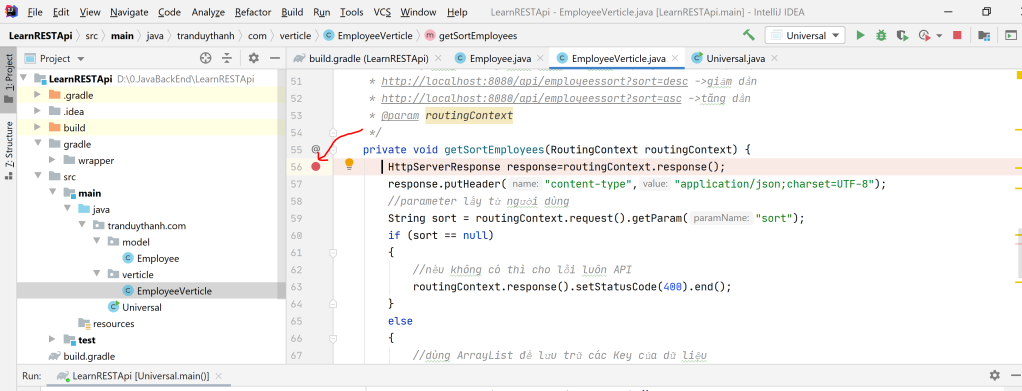
Tiếp theo vào Universal để chạy, nhưng lần này chạy debug chứ không phải run:
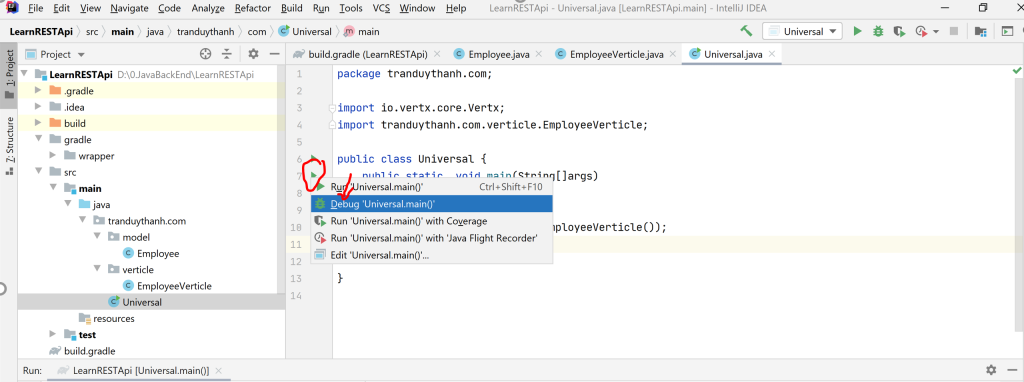
Chọn Debug ‘Universal.main()’. Phần mềm sẽ được đưa vào chế độ chạy debug.
Bây giờ có bất kỳ thao tác triệu gọi API sắp xếp thì nó sẽ tự động vào breakpoint mà mình đã đặt, từ chỗ này ta có thể dễ dàng kiểm tra được dữ liệu parameter đầu vào cũng như quá trình phần mềm xử lý sắp xếp:
Ví dụ:
Bước 1) từ Postman ta triệu gọi API lấy danh sách Employee và sắp xếp giảm dần:
gọi phương thức GET cho API: http://localhost:8080/api/employeessort?sort=desc
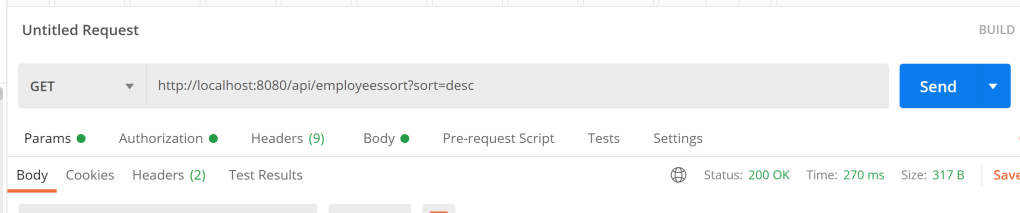
nhấn Send, lúc này Server sẽ cho debug ở API http://localhost:8080/api/employeessort?sort=desc.
Ngay lập tức bên API sẽ di chuyển vào trang thái debug, nơi mà ta đặt breakpoint (dòng 56)

Để di từng dòng lệnh ta nhấn phím F8: Chỉ thị lệnh sẽ đi lần lượt xuống dưới, lúc này ta có thể kiểm tra được giá trị của các parameter mà ta truyền vào khi triệu gọi API:
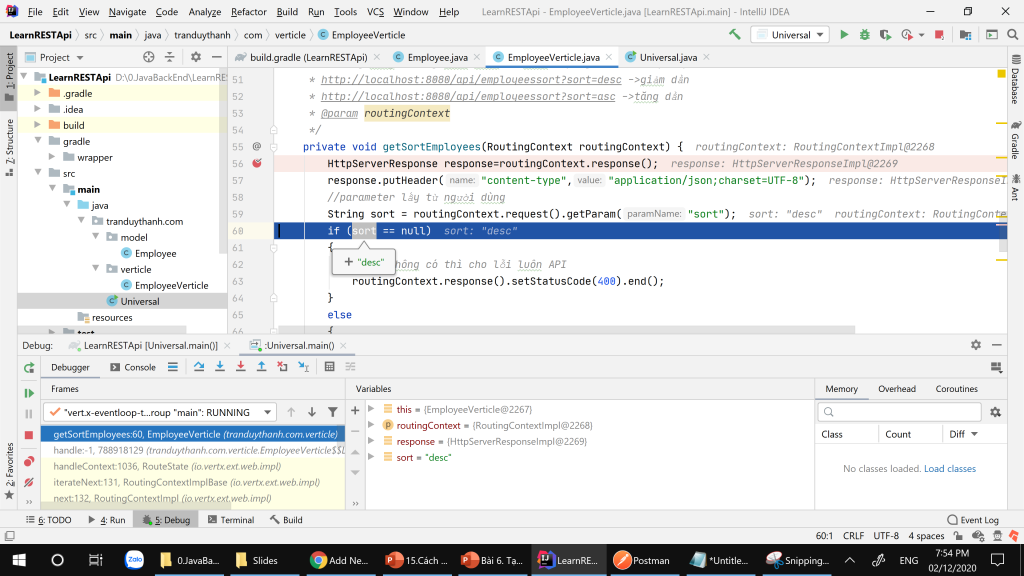
Muốn chạy luôn, dừng Debug thì nhấn phím F9, lúc này ta sẽ thấy được kết quả bên PostMan do API trả về:

Như vậy Tui đã trình bày xong cách viết REST API HTTPGET để lấy danh sách Employee có sắp xếp dữ liệu. các bạn nhớ làm lại nhiều lần để có thể hiểu và triển khai được nhé.
Đây là coding của bài này: https://www.mediafire.com/file/us6jnpsrhjh1ue8/LearnRESTApi_P2.rar/file
chúc các bạn thành công
Hẹn gặp các bạn ở bài tiếp theo, bài lấy chi tiết thông tin của Employee khi biết Id của họ.
One thought on “Bài 6.Tạo Rest API HTTPGET lấy danh sách dữ liệu bằng Vert.X – phần 2”